PIL (Python Image Library) 是 Python 平台处理图片的事实标准,兼具强大的功能和简洁的 API
新建一个 Image
类的实例
PIL 的主要功能定义在 Image
类当中,而 Image
类定义在同名的 Image
模块当中。使用 PIL 的功能,一般都是从新建一个 Image
类的实例开始。新建 Image
类的实例有多种方法。你可以用 Image
模块的 open()
函数打开已有的图片档案,也可以处理其它的实例,或者从零开始构建一个实例。
from PIL import Image
sourceFileName = "source.png"
img = Image.open(sourceFileName)
上述代码引入了 Image 模块,并以 open()
方法打开了 source.png
这个图像,构建了名为 avatar
的实例。如果打开失败,则会抛出 IOError
异常。
接下来你可以使用 show()
方法来查看实例。注意,PIL 会将实例暂存为一个临时文件,而后打开它。
img.show()
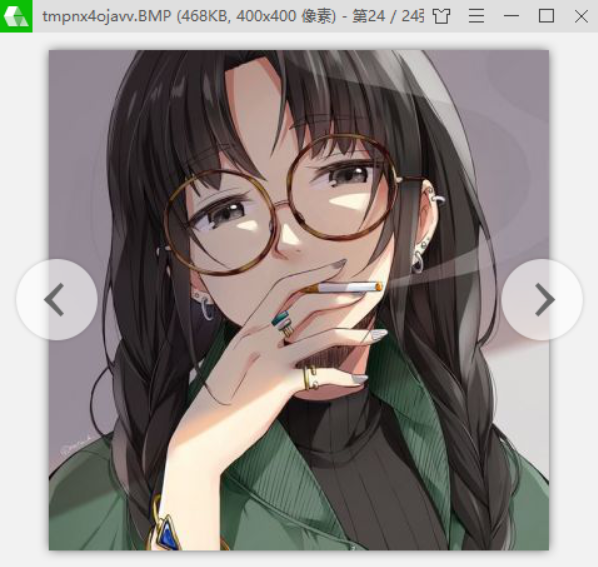
查看实例的属性
Image
类的实例有 5 个属性,分别是:
format
: 以string
返回图片档案的格式(JPG
,PNG
,BMP
,None
, etc.);如果不是从打开文件得到的实例,则返回None
。mode
: 以string
返回图片的模式(RGB, CMYK, etc.);完整的列表参见 官方说明·图片模式列表size
: 以二元 tuple 返回图片档案的尺寸 (width, height)palette
: 仅当mode
为P
时有效,返回ImagePalette
示例info
: 以字典形式返回示例的信息
print(avatar.format, avatar.size, avatar.mode)
# JPEG (400, 400) RGB
看到返回了图片的格式 JPEG
、图片的大小 (400, 400)
和图片的模式 RGB
。
转换 Image 格式为 numpy 数组 array 格式
转换 Image 格式为 numpy 数组 array 格式,进行图像处理
上面读出的 img 还是Image格式,不能对其直接处理,需要对其转换为array格式
这里需要注意的是未转换格式之前 img.size
为(width,height),转换之后img.shape
为(height,width,3)
img = np.array(img, dtype = np.uint8)
实例的方法
Image
类定义了许多方法,这里无法一一列出(也无必要)。如果有需要,可以参看 官方说明·Image
模块。
图片 IO - 转换图片格式
Image
模块提供了 open()
函数打开图片档案,Image
类则提供了 save()
方法将图片实例保存为图片档案。
save()
函数可以以特定的图片格式保存图片档案。比如 save('target.jpg', 'JPG')
将会以 JPG
格式将图片示例保存为 target.jpg
。不过,大多数时候也可以省略图片格式。此时,save()
方法会根据文件扩展名来选择相应的图片格式。
import os, sys
from PIL import Image
for infile in sys.argv[1:]:
f, e = os.path.splitext(infile)
outfile = f + ".jpg"
if infile != outfile:
try:
Image.open(infile).save(outfile)
except IOError:
print "cannot convert", infile
这里,f
是除去扩展名之外的文件名。在 try
语句中,我们尝试打开图片档案,然后以 .jpg
为扩展名保存图片档案。save()
方法会根据扩展名,将图片以 JPG
格式保存为档案。如果图片档案无法打开,则在终端上打印无法转换的消息。
制作缩略图
Image
类的 thumbnail()
方法可以用来制作缩略图。它接受一个二元数组作为缩略图的尺寸,然后将示例缩小到指定尺寸。
import os, sys
from PIL import Image
for infile in sys.argv[1:]:
outfile = os.path.splitext(infile)[0] + ".thumbnail"
if infile != outfile:
try:
im = Image.open(infile)
x, y = im.size
im.thumbnail((x//2, y//2))
im.save(outfile, "JPEG")
except IOError:
print "cannot create thumbnail for", infile
这里我们用 im.size
获取原图档的尺寸,然后以 thumbnail()
制作缩略图,大小则是原先图档的四分之一。同样,如果图档无法打开,则在终端上打印无法执行的提示。
缩放图像
img = Image.open(os.path.join('images', '000906' + '.jpg'))
img_resized = img.resize((width,height)) #和读入图像的格式相同,也是先width后height
#如果想改变插值算法,可以如下(默认为NEAREST)
im_resized = im.resize((width,height), Image.BILINEAR)
NEAREST
:最近滤波。从输入图像中选取最近的像素作为输出像素。它忽略了所有其他的像素。BILINEAR
:双线性滤波。在输入图像的 2x2 矩阵上进行线性插值。注意:PIL的当前版本,做下采样时该滤波器使用了固定输入模板。-
BICUBIC
:双立方滤波。在输入图像的 4x4 矩阵上进行立方插值。注意:PIL 的当前版本,做下采样时该滤波器使用了固定输入模板。 ANTIALIAS
:平滑滤波。这是 PIL 1.1.3版本中新的滤波器。对所有可以影响输出像素的输入像素进行高质量的重采样滤波,以计算输出像素值。在当前的 PIL 版本中,这个滤波器只用于改变尺寸和缩略图方法。
转化为灰度图
gray=img.convert('L')
使用函数 convert()
来进行转换,它是图像实例对象的一个方法,接受一个 mode
参数,用以指定一种色彩模式,mode
的取值可以是如下几种:
1
(1-bit pixels, black and white, stored with one pixel per byte)L
(8-bit pixels, black and white)P
(8-bit pixels, mapped to any other mode using a colour palette)RGB
(3x8-bit pixels, true colour)RGBA
(4x8-bit pixels, true colour with transparency mask)CMYK
(4x8-bit pixels, colour separation)YCbCr
(3x8-bit pixels, colour video format)I
(32-bit signed integer pixels)F
(32-bit floating point pixels)
将灰度图转换为array格式,此时大小为 (height,width), 如果是彩色图像,为 (height,width,3)
gray = np.array(img, dtype = np.uint8)
参考